If you’re looking to insert data into a Room database in Android using Kotlin, this guide is for you! Room is a popular SQLite-based database library that provides an easy-to-use and flexible solution for managing local data in your Android apps.
Let’s dive in!
Prerequisites
First, make sure you have the following prerequisites in place:
- Familiarity with Kotlin programming language and basic Android development concepts.
- Room library added to your project (you can add it via Gradle or Maven). For example, for Gradle, include this line in your
build.gradle
file:<h2> implementation "androidx.room:room-runtime:2.3.0"</h2> <h2> kapt "androidx.room:room-compiler:2.3.0"</h2>
Setting Up Your Data Model
Create a new Kotlin data class that represents the entity you want to store in your database. For instance, let’s assume we are going to create an AppDatabase
and insert a User
entity.
@Entity(tableName "user")
data class User(
<h2> @PrimaryKey(autoGenerate true) val id: Int?,</h2>
<h2> val name: String,</h2>
<h2> val age: Int</h2>
)
Defining Your DAO (Data Access Object)
Create an interface for the Data Access Object (DAO), which will define methods to interact with the database. For our example, let’s create a UserDao
.
<h2>@Dao</h2>
interface UserDao {
@Insert(onConflict OnConflictStrategy.<h2>REPLACE)</h2>
<h2> suspend fun insertUser(user: User): Long</h2>
}
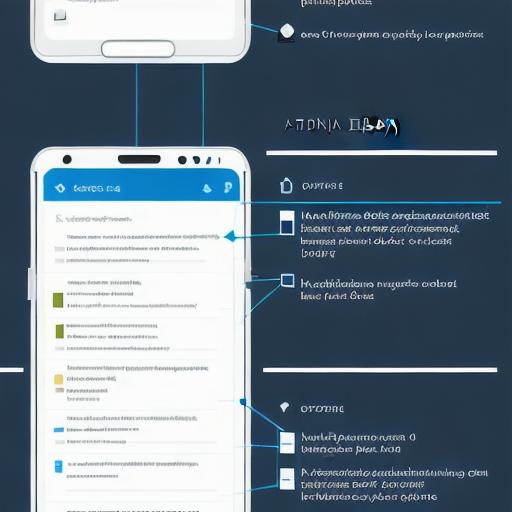
Creating Your Database and DAO Component
Create a companion object inside your AppDatabase
class to instantiate the Room database using the Room.databaseBuilder()
method, passing in your App’s context and your UserDao
.
<h2>@Database(entities [User::class], version 1)</h2>
abstract class AppDatabase : RoomDatabase() {
<h2> abstract fun userDao(): UserDao</h2>
companion object {
<h2> @Volatile</h2>
<h2> private var INSTANCE: AppDatabase? </h2>null
<h2> fun getDatabase(context: Context): AppDatabase {</h2>
<h2> return INSTANCE ?: synchronized(this) {</h2>
val instance Room.databaseBuilder(
context.applicationContext,
<h2> AppDatabase::class.java,</h2>
"app_database"
)
.build()
INSTANCE instance
instance
}
}
}
}
Inserting Data into Your Database
Finally, to insert data into your database, call the UserDao
‘s insertUser()
method and pass in an instance of your User class.
suspend fun insertUser(user: User) {
val db AppDatabase.getDatabase(context).also {
it.open()
}
val userDao it.userDao()
userDao.insertUser(user)
}
Conclusion
In this guide, we learned how to insert data into a Room database using Android Kotlin. We covered the prerequisites, setting up our data model, defining a DAO, creating the database and DAO component, and finally, inserting data into the database.